Beginner’s Guide to Training Your First Image Classifier
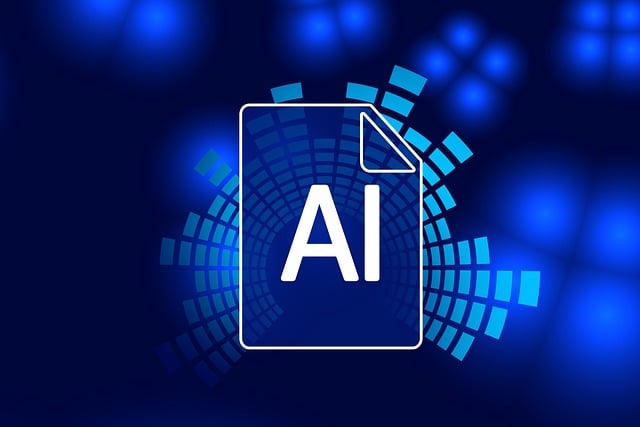
Image classifiers, powered by artificial intelligence (AI), have become an essential tool in various industries, from healthcare to e-commerce. For beginners, training an image classifier may seem daunting, but with the right guidance and tools, you can create a functional model to classify images effectively. This guide walks you through the process step-by-step, making it accessible even for those new to AI and machine learning (ML).
Table of Contents
What Is an Image Classifier?
An image classifier is a machine learning model designed to identify and categorize objects in images. For instance, an image classifier can determine whether an image contains a cat, a dog, or another object.
How Does It Work?
The process involves:
- Input: Feeding labeled images into the model.
- Feature Extraction: Identifying patterns in the images.
- Prediction: Classifying new, unseen images based on learned patterns.
Applications of Image Classification
Before diving into the technical aspects, understanding the real-world applications of image classification can inspire your learning journey:
- Healthcare: Detecting diseases in medical imaging (e.g., identifying tumors in X-rays).
- Retail: Classifying products in online catalogs.
- Autonomous Vehicles: Recognizing road signs and obstacles.
- Wildlife Conservation: Monitoring species through camera traps.
Tools and Frameworks for Training an Image Classifier
Several tools make building an image classifier accessible, even for beginners. Here are some popular choices:
1. TensorFlow and Keras
- Pros: Beginner-friendly, well-documented, supports transfer learning.
- Use Case: Widely used for creating custom models with pre-trained networks like MobileNet or ResNet.
2. PyTorch
- Pros: Flexible, suitable for research and production.
- Use Case: Ideal for advanced users who want more control over their models.
3. Google’s Teachable Machine
- Pros: No coding required, web-based interface.
- Use Case: Great for absolute beginners experimenting with simple classifiers.
4. Fastai
- Pros: Simplifies PyTorch, excellent for rapid prototyping.
- Use Case: Perfect for quick image classification projects.
5. Scikit-learn
- Pros: Lightweight, suitable for simple datasets.
- Use Case: Ideal for educational purposes or small-scale projects.
Step-by-Step Guide to Training Your First Image Classifier
Step 1: Understand the Problem
Define what you want your classifier to do. Examples include:
- Classifying animals in photos.
- Identifying different types of fruits.
Step 2: Gather and Prepare Data
Collect Images
Gather images representing the categories you want to classify. Sources include:
- Public Datasets: Kaggle, ImageNet, or Google’s Open Images.
- Personal Collection: Capture or download images relevant to your project.
Organize Your Data
Create folders for each class (e.g., “Cats” and “Dogs”) and place corresponding images in them.
Data Augmentation
Enhance your dataset by creating variations of existing images, such as:
- Flipping
- Rotating
- Scaling
Step 3: Choose a Pre-Trained Model
Pre-trained models, like ResNet or Inception, save time by leveraging previously trained weights on large datasets like ImageNet. Transfer learning allows you to adapt these models to your dataset.
Step 4: Code Implementation
Setting Up the Environment
- Install required libraries:
bash
pip install tensorflow numpy matplotlib
2. Import necessary modules in Python:
python
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten
from tensorflow.keras.preprocessing.image import ImageDataGenerator
Preprocessing the Data
- Normalize images to improve model performance.
python
datagen = ImageDataGenerator(rescale=1./255, validation_split=0.2)
train_data = datagen.flow_from_directory(‘data’, target_size=(128, 128), batch_size=32, subset=‘training’)
val_data = datagen.flow_from_directory(‘data’, target_size=(128, 128), batch_size=32, subset=‘validation’)
Building the Model
- Use a simple neural network for beginners:
python
model = Sequential([
Flatten(input_shape=(128, 128, 3)), Dense(128, activation=‘relu’),
Dense(64, activation=‘relu’),
Dense(3, activation=‘softmax’)
])
model.compile(optimizer=‘adam’, loss=‘categorical_crossentropy’, metrics=[‘accuracy’])
Training the Model
- Train your classifier using the prepared data:
python
model.fit(train_data, validation_data=val_data, epochs=10)
Evaluating the Model
- Check accuracy on the validation set:
python
val_loss, val_acc = model.evaluate(val_data)
print(f”Validation Accuracy: {val_acc * 100:.2f}%”)
Step 5: Test and Deploy
Testing
Use unseen images to test your model’s predictions.
bash
import numpy as np
from tensorflow.keras.preprocessing import image
test_img = image.load_img(‘test.jpg’, target_size=(128, 128))
test_img_array = np.expand_dims(test_img, axis=0)
predictions = model.predict(test_img_array)
print(f”Predicted Class: {np.argmax(predictions)}“)
Deployment
Deploy your model using platforms like TensorFlow Serving, Flask, or Streamlit for real-world applications.
Tips for Success
- Start Small: Begin with a small dataset and gradually scale up.
- Leverage Transfer Learning: Use pre-trained models to save time and resources.
- Monitor Overfitting: Use techniques like dropout layers and regularization.
- Experiment and Iterate: Try different architectures and hyperparameters to improve accuracy.
Common Challenges and Solutions
Challenge 1: Insufficient Data
- Solution: Use data augmentation or pre-trained models.
Challenge 2: Low Accuracy
- Solution: Experiment with different learning rates or architectures.
Challenge 3: Slow Training
- Solution: Utilize cloud services like Google Colab with GPU support.
Conclusion
Training your first image classifier may seem challenging, but with a structured approach, it becomes manageable and rewarding. By leveraging tools, frameworks, and best practices outlined in this guide, you can successfully create a model tailored to your needs.